Authorization Code Flow with Rich Authorization Requests (RAR)
Using Rich Authorization Requests (RAR), clients can request and obtain fine-grained authorization data from resource owners, such as end users. Clients can pass RAR data to the Pushed Authorization Request (PAR) endpoint as part of the Authorization Code Flow. To learn more, read Configure Pushed Authorization Requests.
In a traditional OAuth 2.0 flow, when a client requests access to a resource server using scopes, the resource owner grants the client access to those resources. In a Rich Authorization Request, clients can pass an authorization_details
parameter to the /par
endpoint to request more granular permissions than those requested in scopes. This allows for more fine-grained control over resource access for both clients and resource owners, mitigating security risks associated with over-provisioning access.
Because Auth0 only supports validating authorization_details
types, you must implement validation for the JSON objects in authorization_details
. To learn more, read Configure Rich Authorization Requests.
How it works
In a Rich Authorization Request, the authorization_details
parameter is a JSON array of objects, each of which must include a type
field represented as a string. The type
field determines the customizable object fields. An authorization_details
array may contain multiple entries of the same type.
The following example for a Rich Authorization Request is of type money_transfer
. It contains the following object fields:
instructedAmount
: The amount of money in USD to be transferred.sourceAccount
: The source bank account from which the money will be transferred.destinationAccount
: The destination bank account to which the money will be transferred.beneficiary
: The recipient of the money transfer.subject
: The subject line of the money transfer.
curl --location 'https://$tenant/oauth/par' \
--request POST \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'client_id=$client_id' \
--data-urlencode 'client_secret=$client_secret' \
--data-urlencode 'redirect_uri=https://jwt.io' \
--data-urlencode 'audience=urn:my-api' \
--data-urlencode 'response_type=code' \
--data-urlencode 'authorization_details=[{"type": "money_transfer", "instructedAmount": {"amount": 2500, "currency": "USD"}, "sourceAccount": "xxxxxxxxxxx1234", "destinationAccount": "xxxxxxxxxxx9876", "beneficiary": "Hanna Herwitz", "subject": "A Lannister Always Pays His Debts"}]'
Was this helpful?
Auth0 presents the authorization_details
to the user to authorize in a custom consent screen. To learn more, read Set customized consent prompt.
You must pass the authorization_details
parameter to the /par
endpoint, which enables the Auth0 Authorization Server to perform early validation of the type
. The /par
endpoint passes authorization requests on the back channel to avoid sensitive data leaking in the front channel, such as the browser. Once you’ve passed the authorization request to the /par
endpoint, the application will redirect to the /authorize
endpoint and then proceed with the Authorization Code Flow. To learn more, read Authorization Code Flow with PAR.
To complete the Authorization Code Flow, exchange the authorization code at the /oauth/token
endpoint, as in the following example:
POST https://$tenant/oauth/token
Content-Type: application/x-www-form-urlencoded
grant_type=authorization_code&client_id=[CLIENT_ID]&client_secret=[CLIENT_SECRET]&code=[AUTHZ_CODE]&redirect_uri=https://jwt.io
Was this helpful?
When successful, you should receive a response with the access token and authorization_details
array:
{
"access_token": "ey...ZQ",
"expires_in": 86400,
"authorization_details": [
{
"type": "money_transfer",
"instructedAmount": {"amount": 2500, "currency": "USD"},
"sourceAccount": "xxxxxxxxxxx1234",
"destinationAccount": "xxxxxxxxxxx9876",
"beneficiary": "Hanna Herwitz",
"subject": "A Lannister Always Pays His Debts"
}
],
"token_type": "Bearer"
}
Was this helpful?
As part of JWT best practices, the client can use authorization_details
to understand the scope of the authorization granted to it without having to inspect the access token. If the requested audience is an API that requires JWE access tokens, the /oauth/token
endpoint returns a response that omits all object fields except for type
from authorization_details
. Access token claims are unaffected in the response.
{
"iss": "https://my_tenant.auth0.com/",
"sub": "auth0|me",
"aud": "https://myapi.authzero.com",
"iat": 1683661385,
"exp": 1683747785,
"azp": "my_client",
"authorization_details": [
{
"type": "money_transfer",
"instructedAmount": {"amount": 2500, "currency": "USD"},
"sourceAccount": "xxxxxxxxxxx1234",
"destinationAccount": "xxxxxxxxxxx9876",
"beneficiary": "Hanna Herwitz",
"subject": "A Lannister Always Pays His Debts"
}
]
}
Was this helpful?
Configure RAR for the Authorization Code Flow
To configure RAR for a resource server, you need to register authorization_details
types. To learn more, read Configure Rich Authorization Requests.
For the Authorization Code Flow, you need to do additional configurations:
Prerequisites
Before configuring Rich Authorization Requests for a resource server during the Authorization Code Flow, you must:
Create a custom domain.
Create a custom Universal Login Pages template. To learn how to customize a Universal Login Page template, read the Page templates API documentation.
Set customized consent prompt
You can render the authorization_details
of a Rich Authorization Request in the consent prompt. To do so, configure the customized-consent
prompt with the appropriate template partials.
In the following PUT
request, configure the customized consent partials:
curl --location --request PUT "https://$tenant/api/v2/prompts/customized-consent/partials" \
--header "Authorization: Bearer $management_access_token" \
--header "Content-Type: application/json" \
--data '{
"customized-consent": {
"form-content": "<div style=\"font-size: 1.3em; font-weight: bold;\">Operation Details</div><hr style=\"margin: 10px 0;\"><div style=\"margin-bottom: 20px;\"></div><div style=\"font-weight: bold;\">Transaction Type</div><div>{{ transaction.params.authorization_details[0].type }}</div><div style=\"margin-bottom: 20px;\"></div><div style=\"font-weight: bold;\">Amount</div><div>{{ transaction.params.authorization_details[0].instructedAmount.amount }} {{ transaction.params.authorization_details[0].instructedAmount.currency }}</div><div style=\"margin-bottom: 20px;\"></div><div style=\"font-weight: bold;\">Recipient</div><div>{{ transaction.params.authorization_details[0].beneficiary }}</div><div style=\"margin-bottom: 20px;\"></div><div style=\"font-weight: bold;\">Destination Account</div><div>{{ transaction.params.authorization_details[0].destinationAccount }}</div><div style=\"margin-bottom: 20px;\"></div>"
}
}'
Was this helpful?
The customized consent template renders the authorization_details
in the following consent prompt that Auth0 shows to the end user:
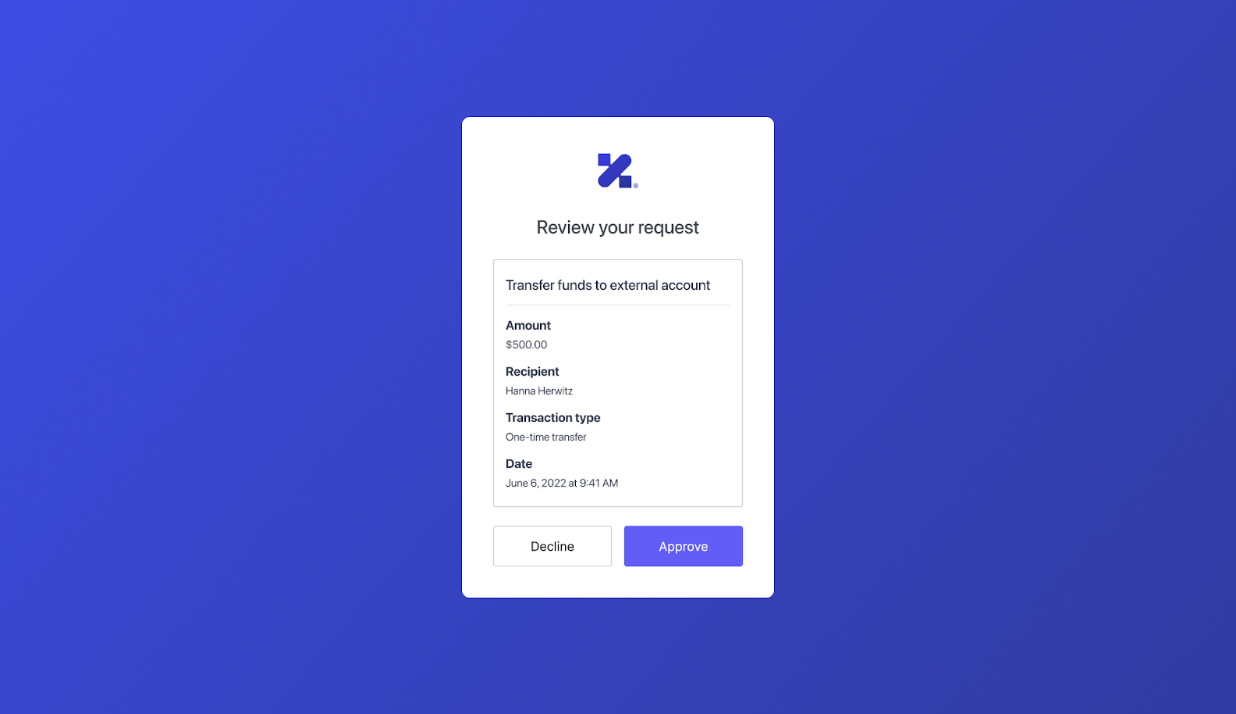
To learn more about how to customize the consent prompt, read:
Access authorization_details in Actions
Auth0 exposes the authorization_details
parameter in the post-login Action via the event.transaction.requested_authorization_details
property. You can reference this property in an Action to show transaction details to the user in an MFA challenge.
What doesn’t Auth0 support?
Auth0 doesn’t support:
Update RAR using Actions.
Advertise RAR types for clients to discover.
Validating RAR objects beyond checking that they have a type property that matches allowed types for the API. For more information, see Configure RAR.