Add Login to Your Next.js Application
This guide demonstrates how to integrate Auth0 with any new or existing Next.js application using the Auth0 Next.js v4 SDK. We recommend that you log in to follow this quickstart with examples configured for your account.
To use Auth0 services, you’ll need to have an application set up in the Auth0 Dashboard. The Auth0 application is where you will configure how you want authentication to work for the project you are developing.
Configure an application
Use the interactive selector to create a new Auth0 application or select an existing application that represents the project you want to integrate with. Every application in Auth0 is assigned an alphanumeric, unique client ID that your application code will use to call Auth0 APIs through the SDK.
Any settings you configure using this quickstart will automatically update for your Application in the Dashboard, which is where you can manage your Applications in the future.
If you would rather explore a complete configuration, you can view a sample application instead.
Configure Callback URLs
A callback URL is a URL in your application that you would like Auth0 to redirect users to after they have authenticated. If not set, users will not be returned to your application after they log in.
Configure Logout URLs
A logout URL is a URL in your application that you would like Auth0 to redirect users to after they have logged out. If not set, users will not be able to log out from your application and will receive an error.
Run the following command within your project directory to install the Auth0 Next.js SDK:
npm i @auth0/nextjs-auth0
The SDK exposes methods and variables that help you integrate Auth0 with your Next.js application using Route Handlers on the backend and React Context with React Hooks on the frontend.
In the root directory of your project, add the file .env.local
with the following environment variables:
AUTH0_SECRET
: A long secret value used to encrypt the session cookie. You can generate a suitable string usingopenssl rand -hex 32
on the command line.APP_BASE_URL
: The base URL of your application.AUTH0_DOMAIN
: The URL of your Auth0 tenant domain. If you are using a Custom Domain with Auth0, set this to the value of your Custom Domain instead of the value reflected in the "Settings" tab.AUTH0_CLIENT_ID
: Your Auth0 application's Client ID.AUTH0_CLIENT_SECRET
: Your Auth0 application's Client Secret.
The SDK will read these values from the Node.js process environment and automatically configure itself.
Create a file at src/lib
/
auth0.ts
. This file provides methods for handling authentication, sessions and user data.
Then, import the Auth0Client
class from the SDK to create an instance and export it as auth0
. This instance is used in your app to interact with Auth0.
Create a file at src/middleware.ts
. This file is used to enforce authentication on specific routes.
The middleware
function intercepts incoming requests and applies Auth0's authentication logic. The matcher
configuration ensures that the middleware runs on all routes except for static files and metadata.
The Landing page src/app/page.tsx
is where users interact with your app. It displays different content based on whether the users is logged in or not.
Edit the file src/app/page.tsx
to add the auth0.getSession()
method to determine if the user is logged in by retrieving the user session.
If there is no user session, the method returns null
and the app displays the Sign up or Log in buttons. If a user sessions exists, the app displays a welcome message with the user's name and a Log out button.
Run this command to start your Next.js development server:
npm run dev
Visit the url http://localhost:3000
in your browser.
You will see:
A Sign up and Log in button if the user is not authenticated.
A welcome message and a Log out button if the user is authenticated.
checkpoint.header
Run Your application.
Verify that your Next.js application redirects you to the Auth0 Universal Login page and that you can now log in or sign up using a username and password or a social provider.
Once that's complete, verify that Auth0 redirects back to your application.
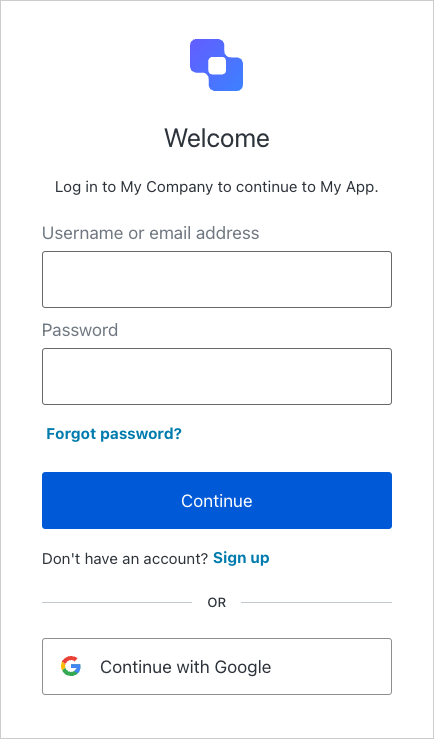
Next Steps
Excellent work! If you made it this far, you should now have login, logout, and user profile information running in your application.
This concludes our quickstart tutorial, but there is so much more to explore. To learn more about what you can do with Auth0, check out:
- Auth0 Dashboard - Learn how to configure and manage your Auth0 tenant and applications
- nextjs-auth0 SDK - Explore the SDK used in this tutorial more fully
- Auth0 Marketplace - Discover integrations you can enable to extend Auth0’s functionality
Sign up for an or to your existing account to integrate directly with your own tenant.